🌳 Vue.js Components
TailAdmin Next.js comes with a variety of pre-designed Next.js + Tailwind components. These components are optimized for server-side rendering (SSR) and static site generation (SSG), ensuring fast loading times and a smooth user experience.
You can do that easily by following this command.
import ComponentName from "../components/ComponentName";
Here are a few examples of the components:
Alert
Alerts are used to provide feedback messages to users.
Preview
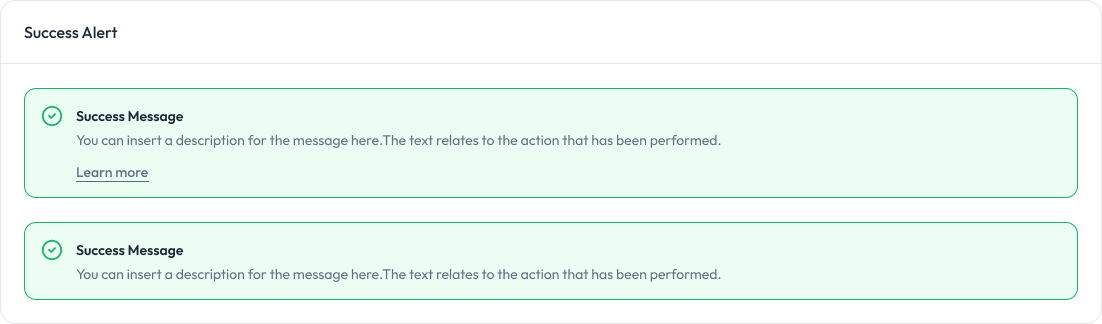
Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
variant | ’success’ | ‘error’ | ‘warning’ | ‘info’ | - | Determines the style and icon of the alert |
title | string | - | The title text of the alert |
message | string | - | The main message content of the alert |
showLink | boolean | false | Whether to show a link in the alert |
linkHref | string | ’#‘ | The URL for the link (if showLink is true) |
linkText | string | ’Learn more’ | The text for the link (if showLink is true) |
Avatar
Avatars are used to display a user’s profile image or initials.
Preview

Props
Prop | Type | Description | Default |
---|---|---|---|
src | string | The URL of the avatar image. | Required |
alt | string | Alt text for the avatar image. | ”User Avatar” |
size | “xsmall” | “small” | “medium” | “large” | “xlarge” | “xxlarge” | Determines the size of the avatar. | ”medium” |
status | “online” | “offline” | “busy” | “none” | Displays a status indicator on the avatar. | ”none” |
Badge
Badges are used to display small status indicators, counts, or labels.
Preview

Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
variant | light | solid | light | The visual style variant of the badge |
size | sm | md | md | The size of the badge |
color | primary | success | error | warning | info | light | dark | primary | The color scheme of the badge |
startIcon | object | - | Icon component to be displayed at the start of the badge |
endIcon | object | - | Icon component to be displayed at the end of the badge |
Breadcrumb
Breadcrumbs are used to display navigation paths and help users track their location within a website.
Preview

Props
Prop | Type | Default | Description |
---|---|---|---|
items | BreadcrumbItem[] | Required | An array of breadcrumb items to be displayed. Each item represents a level in the breadcrumb hierarchy. |
variant | “default” | “withIcon” | “dotted” | “chevron” | “default” | Determines the visual style of the breadcrumb, affecting separators and icon display. |
Button
Button are used to trigger actions, submit forms, or navigate within the application.
Preview

Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
size | sm | md | md | The size of the button |
variant | primary | outline | primary | The visual style variant of the button |
startIcon | object | - | Icon component to be displayed at the start of the button |
endIcon | object | - | Icon component to be displayed at the end of the button |
onClick | () => void | - | Function to be called when the button is clicked |
className | string | ” | Additional CSS classes to be applied to the button |
disabled | boolean | false | Whether the button is disabled |
Button Group
Button groups are used to group related actions together for better organization and user interaction.
Preview

Card
Cards are used to display content and actions related to a single subject in a structured and visually appealing way.
Preview
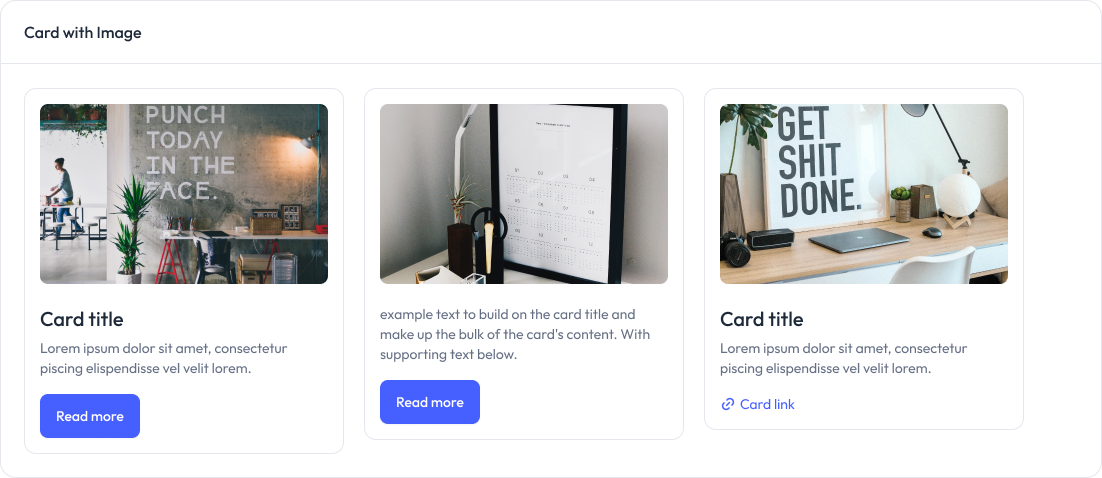
Carousel
Carousels are used to showcase multiple pieces of content in a sliding, interactive format.
Preview
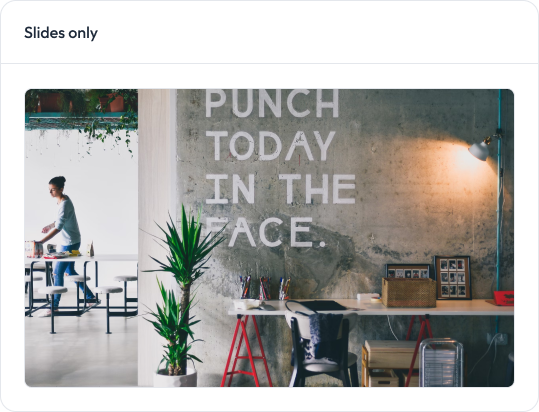
Dropdown
Dropdowns are used to display a list of options or actions in a compact, interactive menu.
Preview
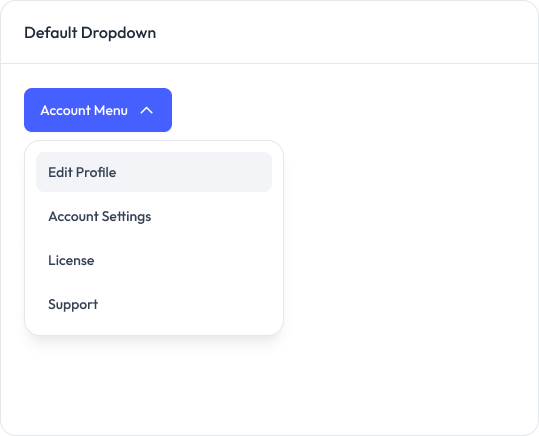
Images
Images are used to display visual content, enhance user experience, and communicate information effectively.
Preview
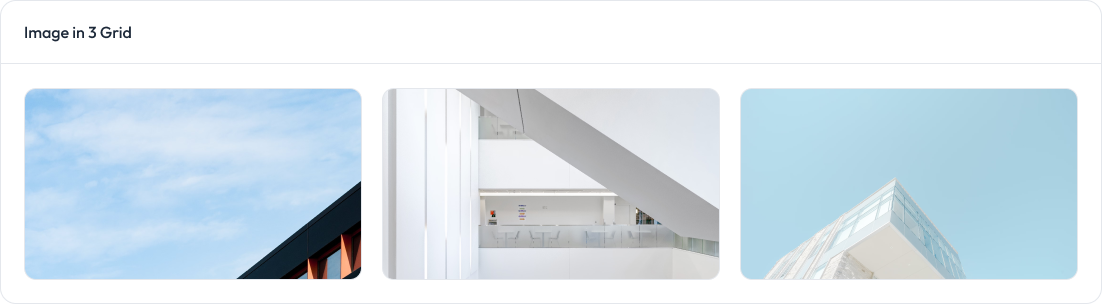
Links
Custom links are used to navigate between pages or perform actions while allowing for customized styles and behaviors.
Preview
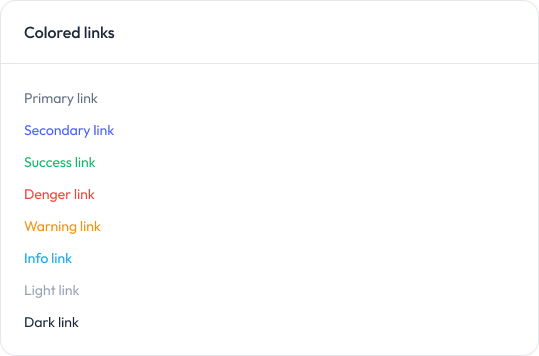
List
Preview
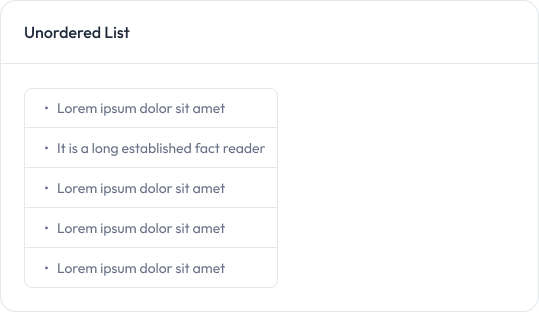
Modal
Modals are used to display content or actions in a popup overlay, typically for focused user interaction without navigating away from the current page.
Preview

Notification
Notifications are used to alert users about important updates, events, or actions in the application.
Preview

Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
type | success | info | warning | error | info | The type of toast notification |
message | string | - | The message to be displayed in the toast |
ProgressBar
Progress bars are used to visually indicate the completion status of a task or process.
Preview

Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
progress | number | - | The progress value (0-100) to be displayed |
size | sm | md | lg | xl | sm | The size of the progress bar |
label | none | outside | inside | none | The position of the progress label |
className | string | ” | Additional CSS classes to be applied to the component |
Pagination
Pagination is used to divide content into separate pages, allowing users to navigate through large datasets or lists efficiently.
Preview

Popover
The Popover component displays floating content next to a trigger element. It’s useful for displaying additional information or controls without cluttering the main interface.
Preview

Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
position | top | right | bottom | left | - | The position of the popover relative to its trigger |
Ribbons
Ribbons are used to highlight or label content with a decorative or attention-grabbing tag, often indicating special status or importance.
Preview
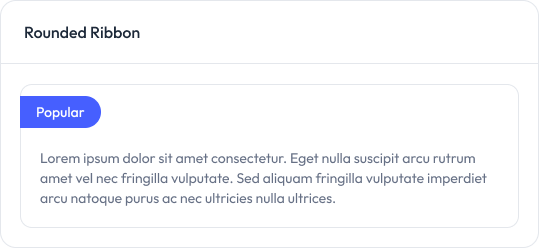
Spinner
Spinners are used to indicate that a process is ongoing or content is loading, providing visual feedback to users.
Preview

Table
The table component is used to display data in a structured, tabular format with customizable rows, columns, and styles.
Preview
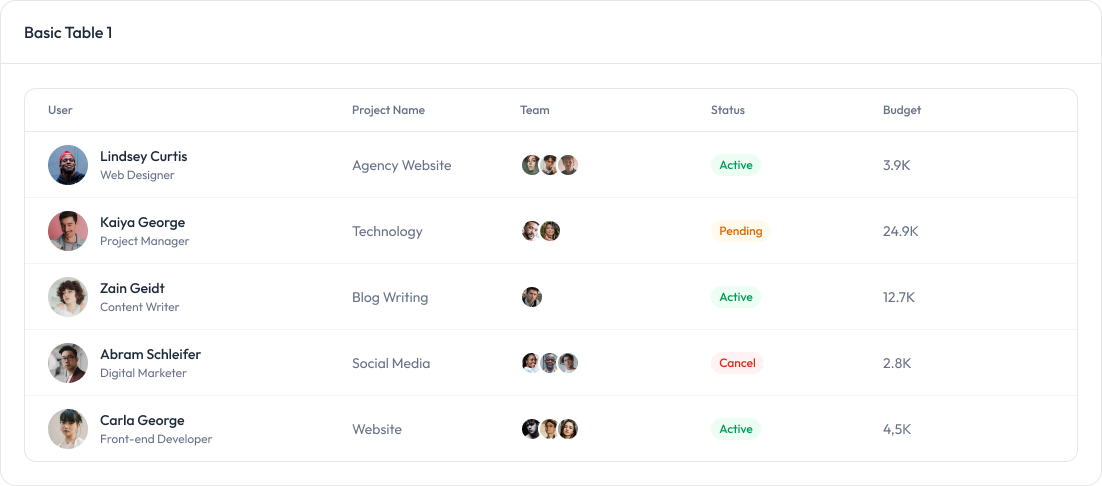
Tabs
Tabs are used to organize content into separate sections, allowing users to switch between different views or categories seamlessly.
Preview
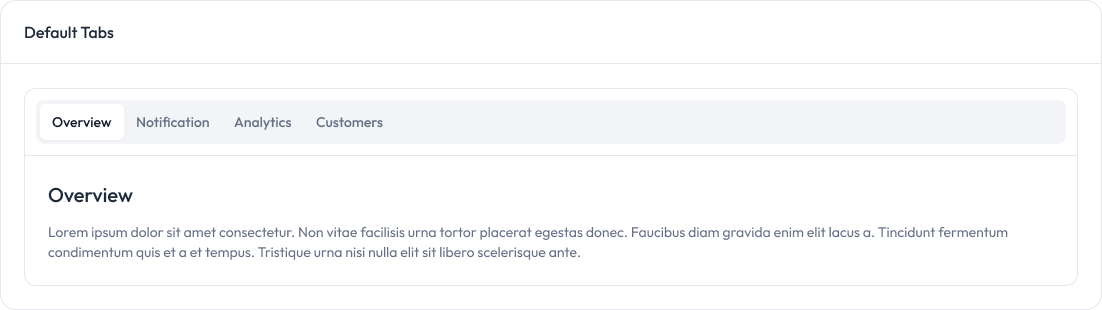
Tooltips
Tooltips are used to provide additional information or hints when a user hovers over or interacts with an element.
Preview
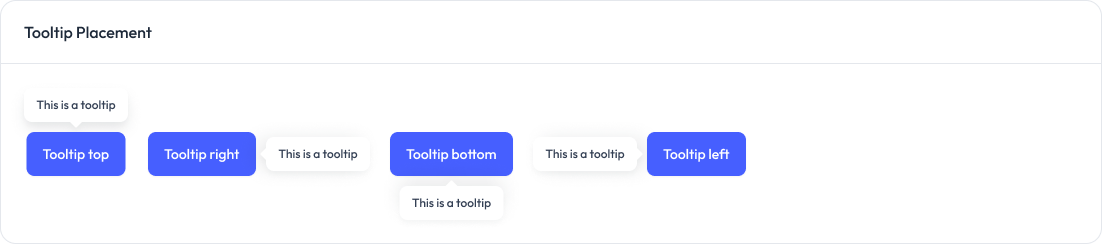
Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
position | top | right | bottom | left | - | The position of the popover relative to its trigger |
Videos
Video components are used to embed and display video content, allowing users to view multimedia within the application.
Preview
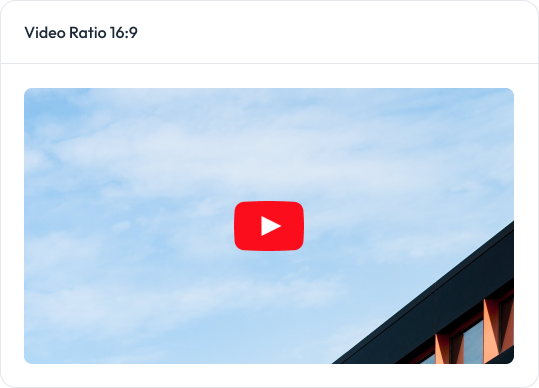
Props
Prop Name | Type | Default Value | Description |
---|---|---|---|
videoId | string | - | The YouTube video ID to be embedded |
aspectRatio | 16:9 | 4:3 | 21:9 | 1:1 | 16:9 | The aspect ratio of the video player |
title | string | YouTube video | The title attribute for the iframe |
className | string | ” | Additional CSS classes to be applied to the component |